728x90
https://www.acmicpc.net/problem/4179
package BKD_0x9_BFS;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.Queue;
import java.util.StringTokenizer;
public class BOJ_4179 {
static char[][] building;
static Queue<Pair> person;
static Queue<Pair> fire;
static int[] dx={1,-1,0,0};
static int[] dy={0,0,1,-1};
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st= new StringTokenizer(br.readLine());
int R = Integer.parseInt(st.nextToken());
int C = Integer.parseInt(st.nextToken());
building = new char[R][C];
person = new LinkedList<>();
fire = new LinkedList<>();
for(int i=0;i<R;i++){
String input = br.readLine();
for(int j=0;j<C;j++){
if(input.charAt(j)=='J'){
person.offer(new Pair(i,j,0));
}
if (input.charAt(j) == 'F') {
fire.offer(new Pair(i,j,0));
}
building[i][j] = input.charAt(j);
}
}
System.out.println(escape(R,C));
}
static String escape(int h,int w){
while (true){
int f_size = fire.size();
int p_size = person.size();
if(p_size==0){
return "IMPOSSIBLE";
}
while (f_size-->0){
Pair fire_location = fire.poll();
for(int i=0;i<4;i++){
int nx = fire_location.x+dx[i];
int ny = fire_location.y+dy[i];
if(nx>=0 && ny>=0 && nx<h && ny<w && (building[nx][ny]=='.' || building[nx][ny]=='V')){
fire.add(new Pair(nx,ny,0));
building[nx][ny]='F';
};
}
}
while(p_size-->0){
Pair person_location = person.poll();
for(int i=0;i<4;i++){
int nx = person_location.x+dx[i];
int ny = person_location.y+dy[i];
if(nx<0 || ny<0 || nx>=h || ny>=w){
return Integer.toString(person_location.time+1);
}
if(nx>=0 && ny>=0 && nx<h && ny<w && building[nx][ny]=='.'){
person.add(new Pair(nx,ny,person_location.time+1));
building[nx][ny]='V';
};
}
}
}
}
static class Pair{
int x;
int y;
int time;
public Pair(int x, int y, int time){
this.x = x;
this.y = y;
this.time = time;
}
}
}
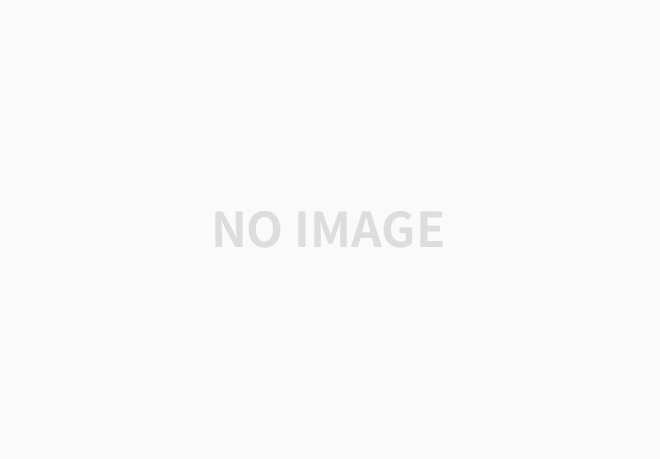
이전에 풀었던 백준5427_불과 크게 달라진 것이 없다고 생각한다. ㅇㅅㅇ
728x90
'🐣 알고리즘 삐약 > 💻 백준 삐약' 카테고리의 다른 글
72 삐약 : 백준 9095| 1,2,3 더하기 [바킹독 문제 풀이|DP|JAVA] (0) | 2024.06.14 |
---|---|
71 삐약 : 백준 1463| 1로만들기 [바킹독 문제 풀이|DP|JAVA] (0) | 2024.06.13 |
69 삐약 : 백준 13913| 숨바꼭질4 [바킹독 문제 풀이|BFS|JAVA] (0) | 2024.06.11 |
68 삐약 : 백준 2573| 빙산 [바킹독 문제 풀이|BFS|JAVA] (1) | 2024.06.09 |
67 삐약 : 백준 5427| 불 [바킹독 문제 풀이|BFS|JAVA] (0) | 2024.06.07 |